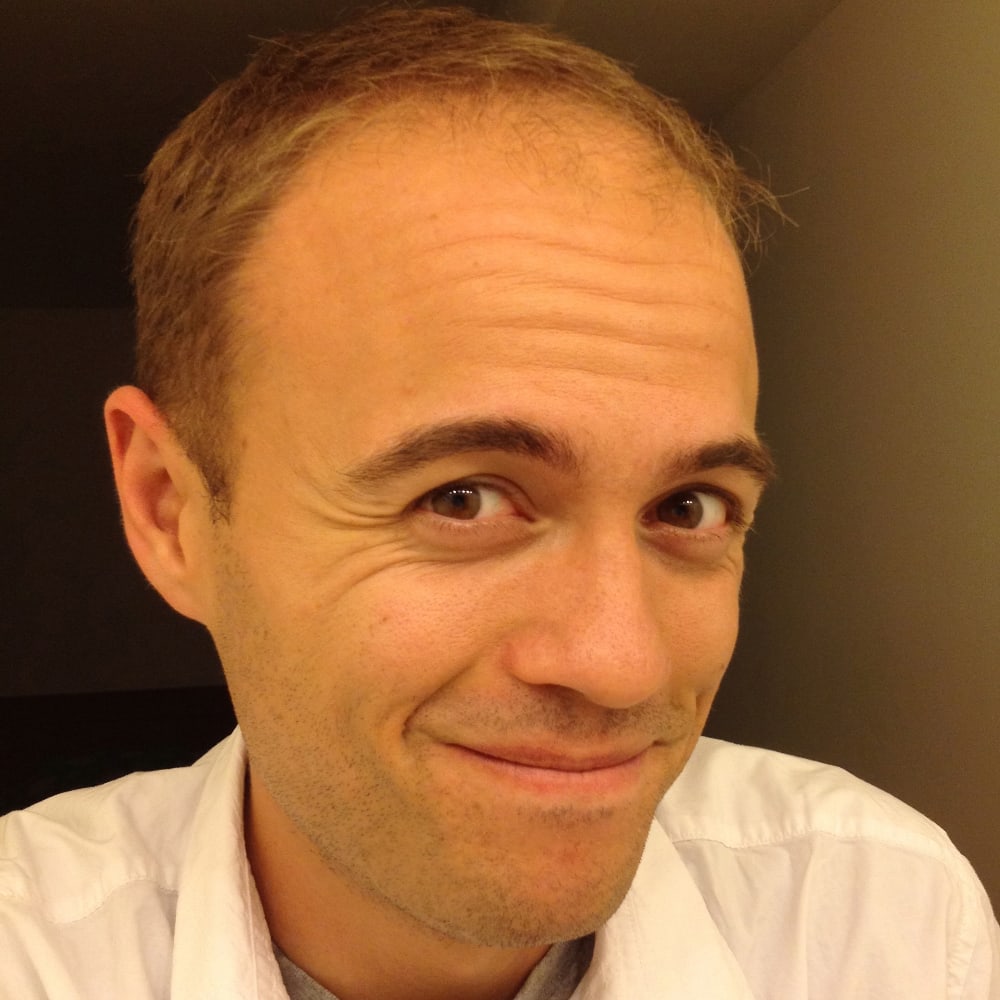
PSA: the "GO" app (prev. JapanTaxi) finally added support last November for account registration with foreign phone numbers, which they'd previously been using to gate the service to Japanese residents.
Strongly recommend using GO over Uber for taxi dispatch in Japan—far better coverage and availability goinc.jp/news/pr/2023/11/10/4zow9wentngt9gjmlsebyn/
Sumibi Yakiniku Kyu
This Yakiniku place in Akita was so fancy that they charge you full price if you cancel within 14 days of your reservation. It's also got a 4.2 on Tabelog. So expectations were quite high… and they were absolutely met. Every plate in the course was perfectly cut, portioned, and arranged. The quality of the meat was great. Our waitress was still in training but was some of the best service I can remember having.
If you're ever in Akita, I strongly encourage calling and making a reservation
Fixing bugs in production when all you have is an iPhone
Noticed an issue with Beckygram yesterday where single-video posts weren't successfully syndicating to Instagram as reels if she didn't also upload a custom thumbnail ("cover") image—which Instagram's API doesn't require.
Even though I'm in Japan with nothing but a phone, a crappy LTE signal from Google Fi (that I can't believe they charge money for), and spotty hotel Wi-Fi, I was glad to find I had the tools to fix it:
- Log into my Mac Studio over SSH using Terminus
- Run
heroku run rails c
to get into the production Rails console to reproduce the error - Clone the repository with Working Copy
- Fix the bug
- Commit & push
- Wait for it to deploy
It was a relief this whole ordeal didn't take more than 15 minutes or so to fix and it's encouraging to know that little one-line bugs won't require me to travel with an iPad or a Mac for supporting her app in production. Nice.
(Oh, and check out her little video of Akita being cute while you're here!)
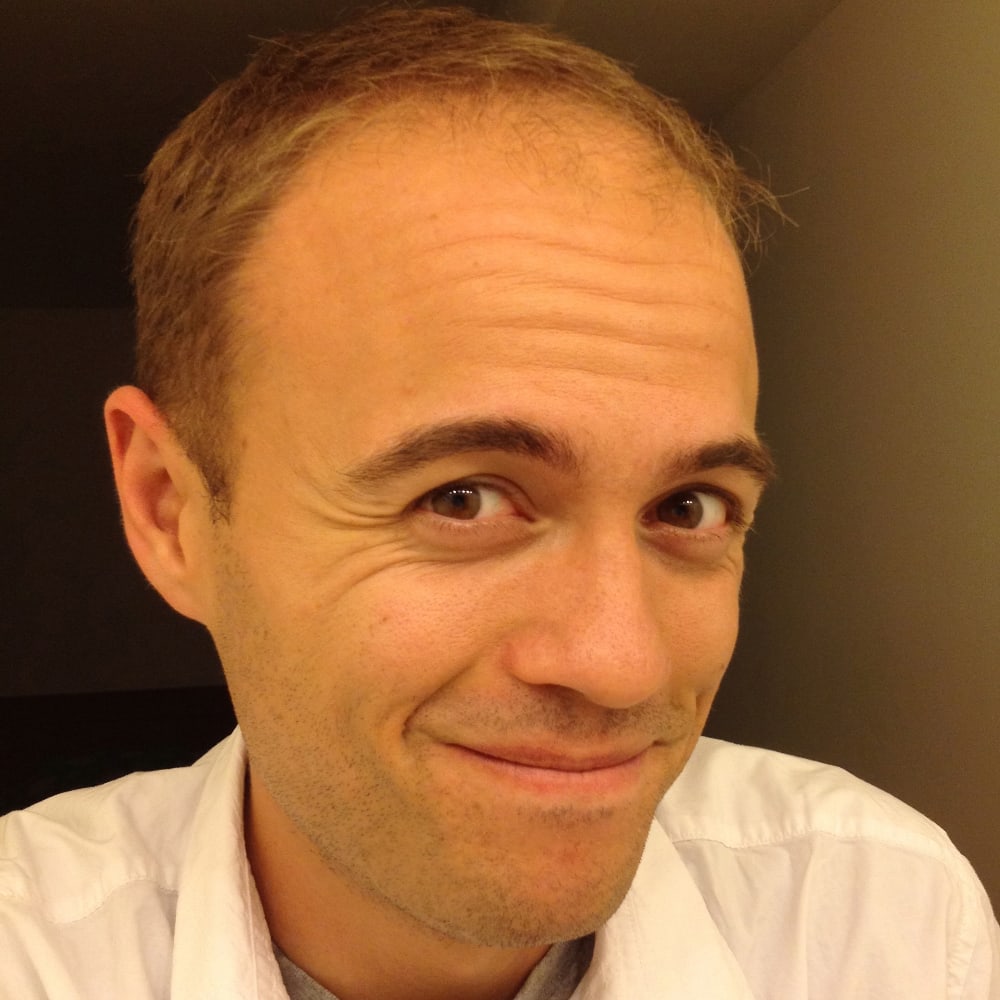
I was extremely skeptical about GM dumping CarPlay and hiring Baris Cetinok from Apple, and after listening to this interview I am almost certain it was a mistake. Dude can barely string together several concepts in a row in comprehensible English. Can't imagine him running a complex software organization theverge.com/24285581/gm-software-baris-cetinok-apple-carplay-android-auto-google-cars-evs-decoder-podcast
Check out this deep-fried egg sandwich
Tonkatsu-fried layered omelette sandwich.
Greatest country on earth. 🇯🇵
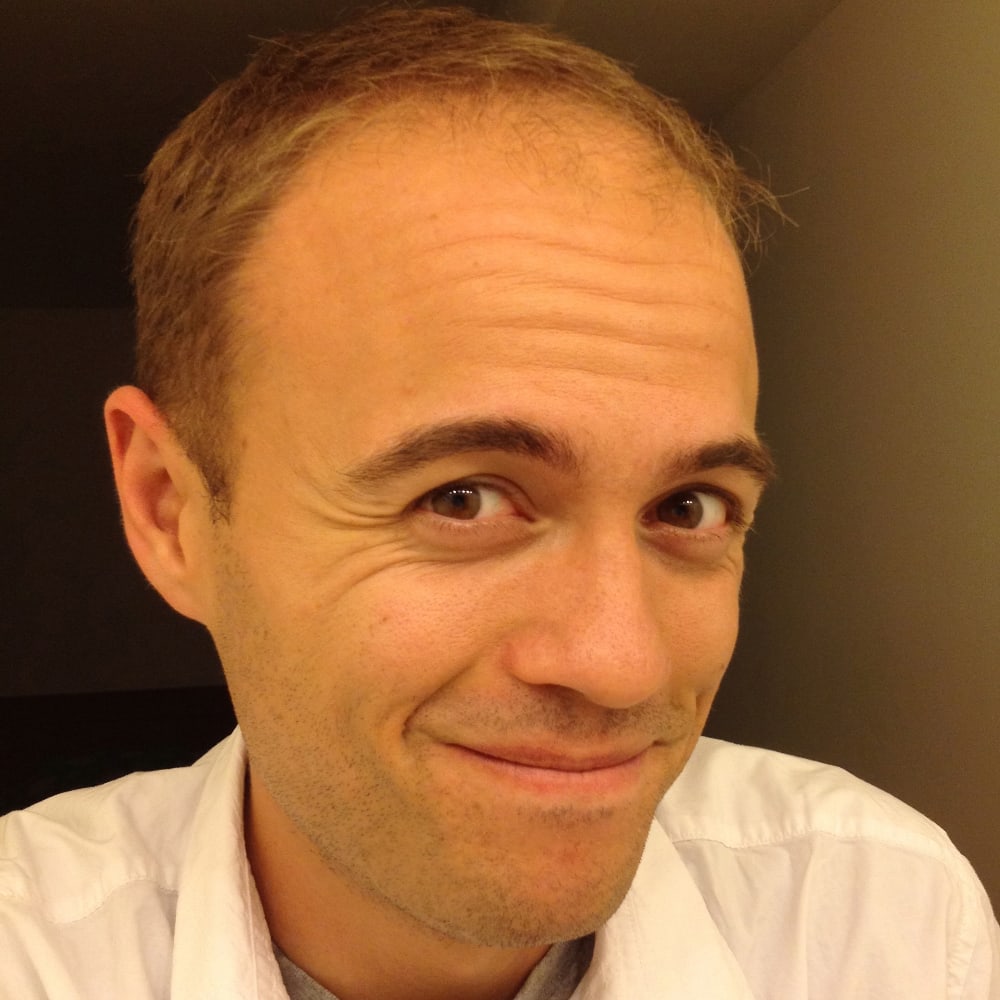
Really nice interview on IndieRails with my better half about her Build with Becky product. Was fun to compare and contrast her recap of year we spent building this thing with my own perspective.
Aside: Becky's got such a great speaking voice that it's a crime that I'm the one with a podcast. Maybe she'll remedy that someday. indierails.com/47
Jikasei Baizen Coffeeann
If you're ever in the mood for a more interesting experience than Starbucks, consider visiting a high-end traditional kissaten. For about $5 out the door had table service for a fantastic hand-ground pour over and the fluffiest, most perfectly buttered toast one can imagine.
TIL that 4 AM is way too goddamn early to record a podcast. Apologies if I'm more chipper than usual, that's probably the coffee talking.
Had some great e-mails this show. You should keep the streak alive and mouth off with your fingers at podcast@searls.co.
Savor this version, folks. Gonna be at least a few weeks until you'll have another one.
It is finished. As mentioned elsewhere, I gave my final conference presentation at Rails World 2024 in Toronto back in September.
The tremendous organizers did me a solid by humoring my request to provide the audio and video feeds they recorded of my talk, which allowed me to create my own edit in the same basic style I've used since discovering screen recording. You can view it on YouTube if you want.
Why watch this one when the official video is also on YouTube? Well, here's what the very exclusive and deluxe and never-before-seen Searls Cut gets you:
- No obstruction, hiding, or movement of the slides themselves—they're the star of the show, not me
- Myself off to the side (where I belong), manually center-tracked with minimal movements to keep me in frame
- Composed in 4K, with slides upscaled to ~1440p
- Same great audio track. I kept in all the umm's and uhh's to humanize me and also because I'm too lazy to bother fixing them
- Native software capture of my slides using Screenflow (as opposed to the conference's HDMI capture)
- Manual removal of the dreaded macOS green dot
- Gently-blurred wide-angle shot as the background instead of cutting between the two video feeds
- Correction of a slide transition where I missed a click on the remote (you can guess, but I'll never tell you which one)
Anyway, if you haven't seen the talk yet, I hope you'll give it a watch. The presentation summarizes a year of my work but it also embeds countless little things life taught me over the 15 years since I started speaking at user groups and regional conferences.
But this chapter of my life has now concluded. I'm excited to be moving on to other things. In the meantime, you can stay tuned to my podcast and subscribe to my newsletter while I get to work.
Apple's own documentation doesn’t know about watchOS 11’s biggest feature
From Apple's support page for connecting an Apple Watch to Wi-Fi:
Note: Apple Watch won’t connect to public networks that require logins, subscriptions, or profiles. These networks, called captive networks, can include free and pay networks in places like businesses, schools, dorms, apartments, hotels, and stores.
This has indeed been my experience ever since buying the Series 0 in 2015. But because the Apple Watch can piggyback off its parent iPhone for data over Bluetooth—and because most people are never more than a few feet from their phone—odds are you've never even noticed that attempting to join a Wi-Fi network with a captive portal would silently fail instead of bringing up a WebKit view.
The people have been clamoring (clamoring!) for a demo of the hot new strength-training system everyone's been talking about, and today Becky has answered their call:
My program, Build with Becky, is designed to make progressive strength training approachable, enjoyable, and sustainable. It’s about helping people get comfortable with lifting, stay consistent, and build confidence using a structured yet mindful, grace-filled approach. 💪
If you've got 3 minutes and a functioning set of eyeballs, I hope you'll give this demo a watch. This video is the cherry on top of several years of work, and I'm incredibly impressed by how well this web app realizes Becky's vision for a more graceful approach to strength training.
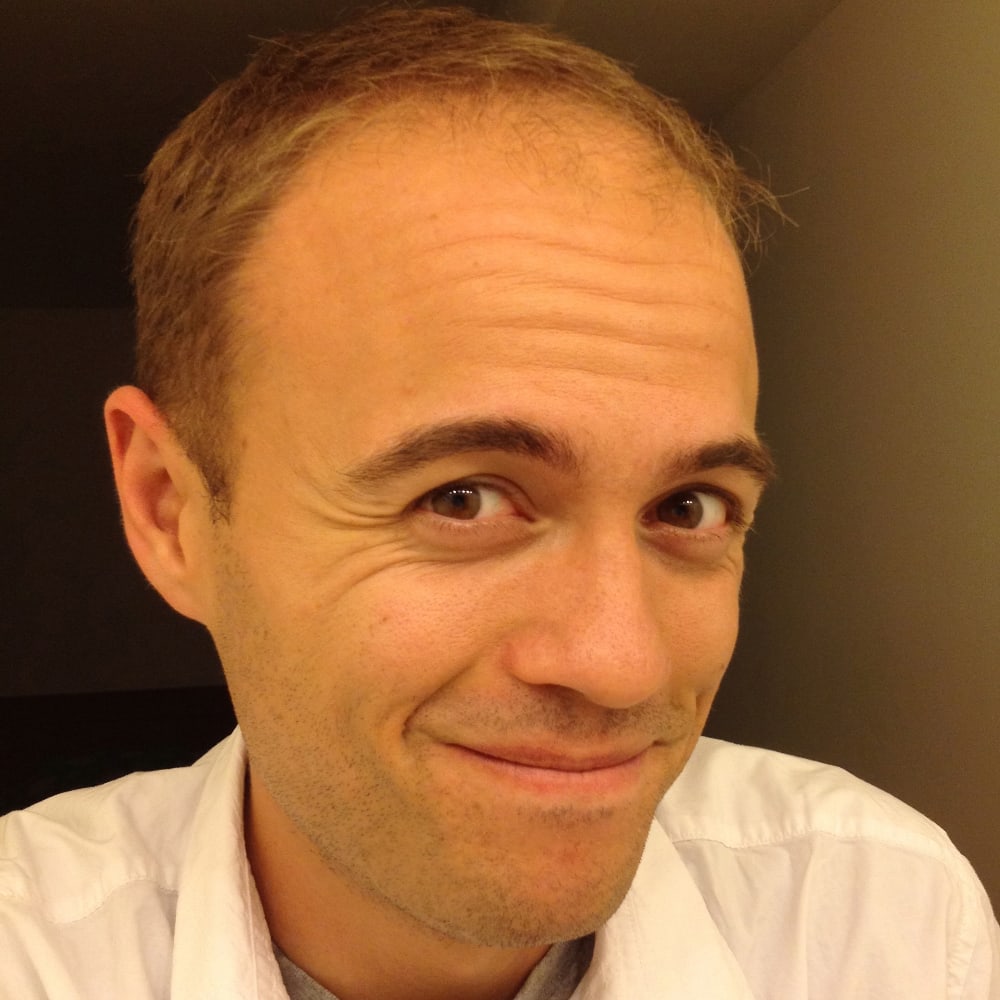
One of the skills I've improved most over the course of my career is Dreading.
I used to dread work that'd actually turn out to be easy or otherwise let a a crushing slog catch me off guard. But now my sense of dread is finely tuned! I was really dreading editing this video and boy was that the correct reaction.
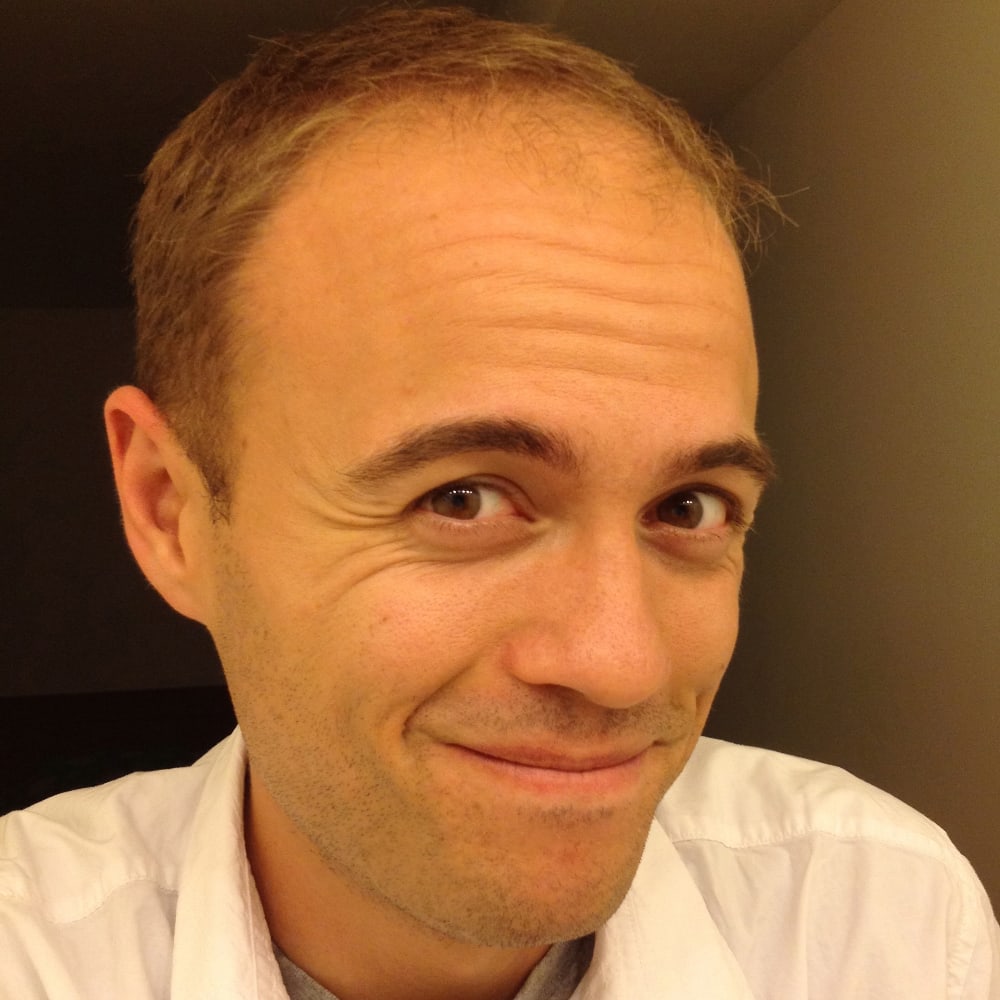
This may surprise some people, because I've been staunchly opposed to the privatization of space exploration my whole life and have been rooting against SpaceX from day one, but I'm a big man… I'm not afraid to admit when I'm wrong.
I've been watching the news, studying the issue closely, and I've changed my position. NASA should award SpaceX as many billions of dollars as it takes to send Elon Musk to Mars as soon as possible.